Transient Biological Pump¶
%load_ext autoreload
%autoreload 2
import os
from itertools import product
import pandas as pd
import numpy as np
import xarray as xr
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
import matplotlib.colors as colors
import cmocean
import cartopy
import cartopy.crs as ccrs
import xpersist as xp
cache_dir = '/glade/p/cgd/oce/projects/cesm2-marbl/xpersist_cache/3d_fields'
if (os.path.isdir(cache_dir)):
xp.settings['cache_dir'] = cache_dir
os.makedirs(cache_dir, exist_ok=True)
os.environ['CESMDATAROOT'] = '/glade/scratch/mclong/inputdata'
import pop_tools
import climo_utils as cu
import utils
import discrete_obs
import plot
import data_collections
ds_grid = pop_tools.get_grid('POP_gx1v7')
masked_area = ds_grid.TAREA.where(ds_grid.REGION_MASK > 0).fillna(0.).expand_dims('region')
masked_area.plot()
/glade/work/mclong/miniconda3/envs/cesm2-marbl/lib/python3.7/site-packages/numba/np/ufunc/parallel.py:365: NumbaWarning: The TBB threading layer requires TBB version 2019.5 or later i.e., TBB_INTERFACE_VERSION >= 11005. Found TBB_INTERFACE_VERSION = 6103. The TBB threading layer is disabled.
warnings.warn(problem)
<matplotlib.collections.QuadMesh at 0x2b15cb9189d0>
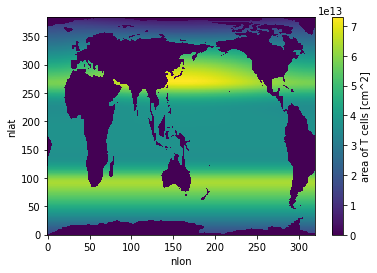
cluster, client = utils.get_ClusterClient()
cluster.scale(12) #adapt(minimum_jobs=0, maximum_jobs=24)
client
Client
|
Cluster
|
experiments = [
'historical',
'SSP1-2.6',
'SSP2-4.5',
'SSP3-7.0',
'SSP5-8.5',
]
time_slice = {
'historical': slice("1990-01-15", "2015-01-15"),
'SSP1-2.6': slice("2086-01-15", "2101-01-15"),
'SSP2-4.5': slice("2086-01-15", "2101-01-15"),
'SSP3-7.0': slice("2086-01-15", "2101-01-15"),
'SSP5-8.5': slice("2086-01-15", "2101-01-15"),
}
varlist = [
'photoC_TOT_zint_100m',
'photoC_diat_zint_100m',
'POC_FLUX_100m',
]
query = dict(
experiment=experiments,
stream='pop.h',
)
ts_int = data_collections.global_integral_timeseries_ann(query)
ts_avg = data_collections.global_mean_timeseries_ann(query)
epoch_mean = data_collections.epoch_mean(query)
epoch_mean
{ 'esm_collection': 'data/campaign-cesm2-cmip6-timeseries.json',
'name': 'epoch_mean',
'operator_kwargs': [ { },
{ }],
'operators': [ 'center_time',
'_mean_time_for_experiment'],
'preprocess': None,
'query': { 'experiment': [ 'historical',
'SSP1-2.6',
'SSP2-4.5',
'SSP3-7.0',
'SSP5-8.5'],
'stream': 'pop.h'}}
nmolcm2s_to_molm2yr = 1e-9 * 1e4 * 86400 * 365.
dsets_epoch = epoch_mean.to_dataset_dict(varlist, clobber=False)
for key, ds in dsets_epoch.items():
for v in varlist:
if ds[v].attrs['units'] == 'mmol/m^3 cm/s':
ds[v] = ds[v] * nmolcm2s_to_molm2yr
ds[v].attrs['units'] = 'mol m$^{-2}$ yr$^{-1}$'
ds['NPP_diat_frac_100m'] = ds.photoC_diat_zint_100m / ds.photoC_TOT_zint_100m
ds['ep_ratio_100m'] = ds.POC_FLUX_100m / ds.photoC_TOT_zint_100m
dsets_epoch
{'ocn.SSP5-8.5.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 3, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates:
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* member_id (member_id) int64 4 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_t_150m (z_t_150m) float32 500.0 1.5e+03 ... 1.45e+04
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
photoC_TOT_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
... ...
HUW (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
salt_to_ppt float64 1e+03
photoC_diat_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
POC_FLUX_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
NPP_diat_frac_100m (member_id, nlat, nlon) float64 nan nan ... nan nan
ep_ratio_100m (member_id, nlat, nlon) float64 nan nan ... nan nan,
'ocn.SSP3-7.0.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 3, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates:
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* member_id (member_id) int64 4 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_t_150m (z_t_150m) float32 500.0 1.5e+03 ... 1.45e+04
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
photoC_TOT_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
... ...
HUW (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
salt_to_ppt float64 1e+03
photoC_diat_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
POC_FLUX_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
NPP_diat_frac_100m (member_id, nlat, nlon) float64 nan nan ... nan nan
ep_ratio_100m (member_id, nlat, nlon) float64 nan nan ... nan nan,
'ocn.SSP2-4.5.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 3, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates:
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* member_id (member_id) int64 4 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_t_150m (z_t_150m) float32 500.0 1.5e+03 ... 1.45e+04
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
photoC_TOT_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
... ...
HUW (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
salt_to_ppt float64 1e+03
photoC_diat_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
POC_FLUX_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
NPP_diat_frac_100m (member_id, nlat, nlon) float64 nan nan ... nan nan
ep_ratio_100m (member_id, nlat, nlon) float64 nan nan ... nan nan,
'ocn.historical.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 11, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates:
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* member_id (member_id) int64 1 2 3 4 5 6 7 8 9 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_t_150m (z_t_150m) float32 500.0 1.5e+03 ... 1.45e+04
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
photoC_TOT_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
... ...
HUW (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
salt_to_ppt float64 1e+03
photoC_diat_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
POC_FLUX_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
NPP_diat_frac_100m (member_id, nlat, nlon) float64 nan nan ... nan nan
ep_ratio_100m (member_id, nlat, nlon) float64 nan nan ... nan nan,
'ocn.SSP1-2.6.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 3, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates:
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* member_id (member_id) int64 4 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_t_150m (z_t_150m) float32 500.0 1.5e+03 ... 1.45e+04
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
photoC_TOT_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
... ...
HUW (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
salt_to_ppt float64 1e+03
photoC_diat_zint_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
POC_FLUX_100m (member_id, nlat, nlon) float64 0.0 0.0 ... 0.0 0.0
NPP_diat_frac_100m (member_id, nlat, nlon) float64 nan nan ... nan nan
ep_ratio_100m (member_id, nlat, nlon) float64 nan nan ... nan nan}
dsets_ts = ts_int.to_dataset_dict(varlist, clobber=False)
for key, ds in dsets_ts.items():
ds['NPP_diat_frac_100m'] = ds.photoC_diat_zint_100m / ds.photoC_TOT_zint_100m
ds['ep_ratio_100m'] = ds.POC_FLUX_100m / ds.photoC_TOT_zint_100m
dsets_ts
{'ocn.SSP5-8.5.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 3, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, time: 86, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates: (12/13)
* member_id (member_id) int64 4 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
... ...
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
* time (time) int64 2015 2016 2017 2018 ... 2098 2099 2100
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
transport_regions (transport_reg) |S384 b'Global Ocean - Marginal S...
... ...
salt_to_ppt float64 1e+03
photoC_TOT_zint_100m (time, member_id) float64 ...
photoC_diat_zint_100m (time, member_id) float64 ...
POC_FLUX_100m (time, member_id) float64 ...
NPP_diat_frac_100m (time, member_id) float64 0.3839 0.3697 ... 0.2938
ep_ratio_100m (time, member_id) float64 0.1475 0.1459 ... 0.1339,
'ocn.SSP3-7.0.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 3, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, time: 86, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates: (12/13)
* member_id (member_id) int64 4 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
... ...
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
* time (time) int64 2015 2016 2017 2018 ... 2098 2099 2100
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
transport_regions (transport_reg) |S384 b'Global Ocean - Marginal S...
... ...
salt_to_ppt float64 1e+03
photoC_TOT_zint_100m (time, member_id) float64 ...
photoC_diat_zint_100m (time, member_id) float64 ...
POC_FLUX_100m (time, member_id) float64 ...
NPP_diat_frac_100m (time, member_id) float64 0.3778 0.3623 ... 0.3396
ep_ratio_100m (time, member_id) float64 0.1462 0.1449 ... 0.1399,
'ocn.SSP2-4.5.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 3, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, time: 86, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates: (12/13)
* member_id (member_id) int64 4 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
... ...
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
* time (time) int64 2015 2016 2017 2018 ... 2098 2099 2100
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
transport_regions (transport_reg) |S384 b'Global Ocean - Marginal S...
... ...
salt_to_ppt float64 1e+03
photoC_TOT_zint_100m (time, member_id) float64 ...
photoC_diat_zint_100m (time, member_id) float64 ...
POC_FLUX_100m (time, member_id) float64 ...
NPP_diat_frac_100m (time, member_id) float64 0.3764 0.3713 ... 0.3498
ep_ratio_100m (time, member_id) float64 0.1466 0.1453 ... 0.1427,
'ocn.historical.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 11, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, time: 165, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates: (12/13)
* member_id (member_id) int64 1 2 3 4 5 6 7 8 9 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
... ...
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
* time (time) int64 1850 1851 1852 1853 ... 2012 2013 2014
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
transport_regions (transport_reg) |S384 b'Global Ocean - Marginal S...
... ...
salt_to_ppt float64 1e+03
photoC_TOT_zint_100m (time, member_id) float64 ...
photoC_diat_zint_100m (time, member_id) float64 ...
POC_FLUX_100m (time, member_id) float64 ...
NPP_diat_frac_100m (time, member_id) float64 0.3471 0.3643 ... 0.3742
ep_ratio_100m (time, member_id) float64 0.1445 0.1477 ... 0.1462,
'ocn.SSP1-2.6.pop.h': <xarray.Dataset>
Dimensions: (lat_aux_grid: 395, member_id: 3, moc_comp: 3, moc_z: 61, nlat: 384, nlon: 320, time: 86, transport_comp: 5, transport_reg: 2, z_t: 60, z_t_150m: 15, z_w: 60, z_w_bot: 60, z_w_top: 60)
Coordinates: (12/13)
* member_id (member_id) int64 4 10 11
* lat_aux_grid (lat_aux_grid) float32 -79.49 -78.95 ... 89.47 90.0
TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8
* z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19
* moc_z (moc_z) float32 0.0 1e+03 2e+03 ... 5.25e+05 5.5e+05
... ...
* z_t (z_t) float32 500.0 1.5e+03 ... 5.125e+05 5.375e+05
ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0
* z_w_bot (z_w_bot) float32 1e+03 2e+03 ... 5.25e+05 5.5e+05
* z_w (z_w) float32 0.0 1e+03 2e+03 ... 5e+05 5.25e+05
ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41
* time (time) int64 2015 2016 2017 2018 ... 2098 2099 2100
Dimensions without coordinates: moc_comp, nlat, nlon, transport_comp, transport_reg
Data variables: (12/58)
nsurface_u float64 8.297e+04
DXU (nlat, nlon) float64 2.397e+06 ... 1.391e+06
DYT (nlat, nlon) float64 5.94e+06 5.94e+06 ... 5.046e+06
vonkar float64 0.4
dz (z_t) float32 1e+03 1e+03 1e+03 ... 2.5e+04 2.5e+04
transport_regions (transport_reg) |S384 b'Global Ocean - Marginal S...
... ...
salt_to_ppt float64 1e+03
photoC_TOT_zint_100m (time, member_id) float64 ...
photoC_diat_zint_100m (time, member_id) float64 ...
POC_FLUX_100m (time, member_id) float64 ...
NPP_diat_frac_100m (time, member_id) float64 0.3775 0.3596 ... 0.3228
ep_ratio_100m (time, member_id) float64 0.1462 0.1446 ... 0.1406}
import seaborn as sns
current_palette = sns.color_palette('colorblind', 8)
sns.palplot(current_palette)
exp_colors = {
'PI': current_palette.as_hex()[-1],
'historical': 'k',
'SSP1-2.6': current_palette.as_hex()[0],
'SSP2-4.5': current_palette.as_hex()[1],
'SSP3-7.0': current_palette.as_hex()[2],
'SSP5-8.5': current_palette.as_hex()[3],
}

variable_labels = dict(
time='Year',
FG_CO2='Air-sea CO$_2$ flux [Pg C yr$^{-1}$]',
photoC_TOT_zint_100m='NPP (z > -100m) [Pg C yr$^{-1}$]',
photoC_TOT_zint='NPP [Pg C yr$^{-1}$]',
POC_FLUX_100m='POC flux (100 m) [Pg C yr$^{-1}$]',
ATM_CO2='Atmospheric CO$_2$ [ppm]',
SST='Temperature [°C]',
ep_ratio_100m='ep ratio',
NPP_diat_frac_100m='Diatom fraction of NPP',
)
varlist_all = varlist + ['NPP_diat_frac_100m', 'ep_ratio_100m']
nrow = 2
ncol = 2
#fig, axs = plt.subplots(nrow, ncol, figsize=(4*ncol, 3*nrow), constrained_layout=False)
fig = plt.figure(figsize=(12, 9))
gs = gridspec.GridSpec(
nrows=2, ncols=2,
hspace=0.25,
wspace=0.22,
)
axs = np.empty((2, 2)).astype(object)
for i, j in product(range(2), range(2)):
axs[i, j] = plt.subplot(gs[i, j])
X = [['time', 'time'], ['time', 'time'],]
Y = [['photoC_TOT_zint_100m', 'POC_FLUX_100m'], ['ep_ratio_100m', 'NPP_diat_frac_100m'],]
for i, j in product(range(2), range(2)):
ax = axs[i, j]
x = X[i][j]
y = Y[i][j]
for exp in experiments:
if exp == 'piControl':
continue
ds = dsets_ts[f'ocn.{exp}.pop.h']
#for member_id in ds.member_id.values:
# ax.plot(ds.time, ds[v].sel(member_id=member_id), '-', alpha=0.25, linewidth=1, color=exp_colors[exp])
ax.plot(ds[x], ds[y].mean('member_id'), '-', linewidth=2, color=exp_colors[exp], label=exp)
ax.set_ylabel(variable_labels[y])
ax.legend()
ax.set_xlabel(variable_labels[x])
utils.label_plots(fig, [ax for ax in axs.ravel()], xoff=-0.03, yoff=0.015)
utils.savefig(f'global-timeseries-bio-pump.pdf')
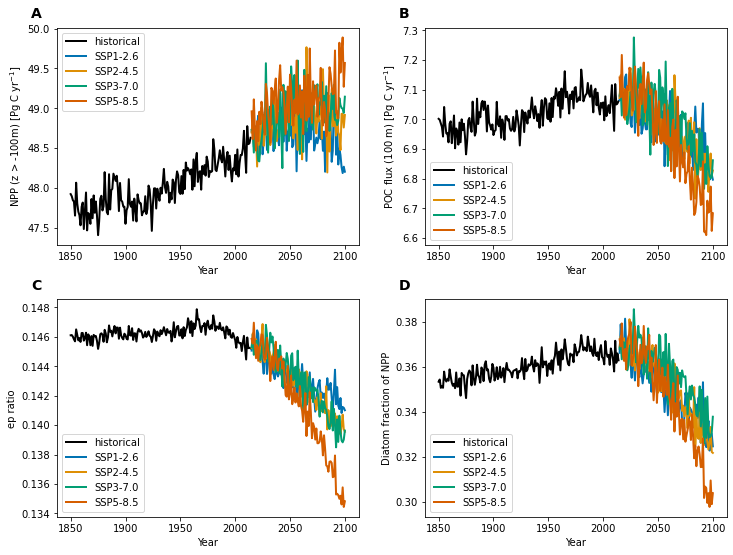
ds_delta = (dsets_epoch['ocn.SSP5-8.5.pop.h'][varlist_all] - dsets_epoch['ocn.historical.pop.h'][varlist_all]).mean('member_id')
ds_delta
<xarray.Dataset> Dimensions: (nlat: 384, nlon: 320) Coordinates: ULONG (nlat, nlon) float64 321.1 322.3 ... 319.6 320.0 ULAT (nlat, nlon) float64 -78.95 -78.95 ... 72.41 72.41 TLONG (nlat, nlon) float64 320.6 321.7 ... 319.4 319.8 TLAT (nlat, nlon) float64 -79.22 -79.22 ... 72.19 72.19 Dimensions without coordinates: nlat, nlon Data variables: photoC_TOT_zint_100m (nlat, nlon) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 photoC_diat_zint_100m (nlat, nlon) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 POC_FLUX_100m (nlat, nlon) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 NPP_diat_frac_100m (nlat, nlon) float64 nan nan nan nan ... nan nan nan ep_ratio_100m (nlat, nlon) float64 nan nan nan nan ... nan nan nan
xarray.Dataset
- nlat: 384
- nlon: 320
- ULONG(nlat, nlon)float64321.1 322.3 323.4 ... 319.6 320.0
- long_name :
- array of u-grid longitudes
- units :
- degrees_east
array([[321.12500894, 322.25000897, 323.375009 , ..., 317.75000884, 318.87500887, 320.0000089 ], [321.12500894, 322.25000897, 323.375009 , ..., 317.75000884, 318.87500887, 320.0000089 ], [321.12500894, 322.25000897, 323.375009 , ..., 317.75000884, 318.87500887, 320.0000089 ], ..., [320.48637802, 320.97240884, 321.4577638 , ..., 319.02760897, 319.51363979, 320.00001324], [320.45160767, 320.90286181, 321.35342745, ..., 319.097156 , 319.54841014, 320.00001293], [320.41397858, 320.82760085, 321.24052915, ..., 319.17241696, 319.58603923, 320.00001259]])
- ULAT(nlat, nlon)float64-78.95 -78.95 ... 72.41 72.41
- long_name :
- array of u-grid latitudes
- units :
- degrees_north
array([[-78.95289509, -78.95289509, -78.95289509, ..., -78.95289509, -78.95289509, -78.95289509], [-78.41865507, -78.41865507, -78.41865507, ..., -78.41865507, -78.41865507, -78.41865507], [-77.88441506, -77.88441506, -77.88441506, ..., -77.88441506, -77.88441506, -77.88441506], ..., [ 71.51215224, 71.51766482, 71.52684191, ..., 71.51766482, 71.51215224, 71.51031365], [ 71.95983548, 71.96504258, 71.97371054, ..., 71.96504258, 71.95983548, 71.95809872], [ 72.4135549 , 72.41841155, 72.42649554, ..., 72.41841155, 72.4135549 , 72.41193498]])
- TLONG(nlat, nlon)float64320.6 321.7 322.8 ... 319.4 319.8
- long_name :
- array of t-grid longitudes
- units :
- degrees_east
array([[320.56250892, 321.68750895, 322.81250898, ..., 317.18750883, 318.31250886, 319.43750889], [320.56250892, 321.68750895, 322.81250898, ..., 317.18750883, 318.31250886, 319.43750889], [320.56250892, 321.68750895, 322.81250898, ..., 317.18750883, 318.31250886, 319.43750889], ..., [320.25133086, 320.75380113, 321.25577325, ..., 318.74424456, 319.24621668, 319.74869143], [320.23459477, 320.70358949, 321.17207442, ..., 318.82794339, 319.29642832, 319.76542721], [320.21650899, 320.6493303 , 321.08163473, ..., 318.91838308, 319.3506875 , 319.78351267]])
- TLAT(nlat, nlon)float64-79.22 -79.22 ... 72.19 72.19
- long_name :
- array of t-grid latitudes
- units :
- degrees_north
array([[-79.22052261, -79.22052261, -79.22052261, ..., -79.22052261, -79.22052261, -79.22052261], [-78.68630626, -78.68630626, -78.68630626, ..., -78.68630626, -78.68630626, -78.68630626], [-78.15208992, -78.15208992, -78.15208992, ..., -78.15208992, -78.15208992, -78.15208992], ..., [ 71.29031715, 71.29408252, 71.30160692, ..., 71.30160692, 71.29408252, 71.29031716], [ 71.73524335, 71.73881845, 71.74596231, ..., 71.74596231, 71.73881845, 71.73524335], [ 72.18597561, 72.18933231, 72.19603941, ..., 72.19603941, 72.18933231, 72.18597562]])
- photoC_TOT_zint_100m(nlat, nlon)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([[0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0.02530587, 0.03092501, 0.02881577, ..., 0. , 0. , 0. ], ..., [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ]])
- photoC_diat_zint_100m(nlat, nlon)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([[0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0.00463665, 0.00535796, 0.00480258, ..., 0. , 0. , 0. ], ..., [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ]])
- POC_FLUX_100m(nlat, nlon)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([[0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0.02229476, 0.02500899, 0.02668867, ..., 0. , 0. , 0. ], ..., [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ], [0. , 0. , 0. , ..., 0. , 0. , 0. ]])
- NPP_diat_frac_100m(nlat, nlon)float64nan nan nan nan ... nan nan nan nan
array([[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [-0.11855408, -0.12664945, -0.11823797, ..., nan, nan, nan], ..., [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]])
- ep_ratio_100m(nlat, nlon)float64nan nan nan nan ... nan nan nan nan
array([[ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [-1.16435834, -0.94498757, -1.19052237, ..., nan, nan, nan], ..., [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan], [ nan, nan, nan, ..., nan, nan, nan]])
dsp = utils.pop_add_cyclic(ds_delta)
dsp
<xarray.Dataset> Dimensions: (nlat: 384, nlon: 321) Dimensions without coordinates: nlat, nlon Data variables: TLAT (nlat, nlon) float64 -79.22 -79.22 ... 80.31 80.31 TLONG (nlat, nlon) float64 -220.6 -219.4 ... -39.57 -39.86 photoC_TOT_zint_100m (nlat, nlon) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 photoC_diat_zint_100m (nlat, nlon) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 POC_FLUX_100m (nlat, nlon) float64 0.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 NPP_diat_frac_100m (nlat, nlon) float64 nan nan nan nan ... nan nan nan ep_ratio_100m (nlat, nlon) float64 nan nan nan nan ... nan nan nan
xarray.Dataset
- nlat: 384
- nlon: 321
- TLAT(nlat, nlon)float64-79.22 -79.22 ... 80.31 80.31
array([[-79.22052261, -79.22052261, -79.22052261, ..., -79.22052261, -79.22052261, -79.22052261], [-78.68630626, -78.68630626, -78.68630626, ..., -78.68630626, -78.68630626, -78.68630626], [-78.15208992, -78.15208992, -78.15208992, ..., -78.15208992, -78.15208992, -78.15208992], ..., [ 81.44584238, 81.44584238, 81.44466079, ..., 81.44229771, 81.44466079, 81.44584238], [ 80.87803543, 80.87803543, 80.87705778, ..., 80.87510244, 80.87705778, 80.87803543], [ 80.31321311, 80.31321311, 80.31241206, ..., 80.31080987, 80.31241206, 80.31321311]])
- TLONG(nlat, nlon)float64-220.6 -219.4 ... -39.57 -39.86
array([[-220.56249613, -219.43749609, -218.31249606, ..., 137.18750382, 138.31250385, 139.43750388], [-220.56249613, -219.43749609, -218.31249606, ..., 137.18750382, 138.31250385, 139.43750388], [-220.56249613, -219.43749609, -218.31249606, ..., 137.18750382, 138.31250385, 139.43750388], ..., [ -39.7932723 , -40.20670806, -40.62006565, ..., -38.96679888, -39.37991654, -39.79327229], [ -39.82753619, -40.17244447, -40.51730173, ..., -39.13798089, -39.48268046, -39.82753618], [ -39.85740526, -40.14257567, -40.42771349, ..., -39.28723255, -39.5722687 , -39.85740525]])
- photoC_TOT_zint_100m(nlat, nlon)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]])
- photoC_diat_zint_100m(nlat, nlon)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]])
- POC_FLUX_100m(nlat, nlon)float640.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]])
- NPP_diat_frac_100m(nlat, nlon)float64nan nan nan nan ... nan nan nan nan
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], ..., [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]])
- ep_ratio_100m(nlat, nlon)float64nan nan nan nan ... nan nan nan nan
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], ..., [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]])
fig = plt.figure(figsize=(12, 6))
prj = ccrs.Robinson(central_longitude=305.0)
titles = dict(
photoC_TOT_zint_100m='NPP',
POC_FLUX_100m='POC export (100m)',
ep_ratio_100m='Export ratio',
NPP_diat_frac_100m='Fraction of NPP by diatoms',
)
nrow, ncol = 2, 2
gs = gridspec.GridSpec(
nrows=nrow, ncols=ncol*3,
width_ratios=(1, 0.02, 0.02)*ncol,
wspace=0.15,
hspace=0.1,
)
axs = np.empty((nrow, ncol)).astype(object)
caxs= np.empty((nrow, ncol)).astype(object)
for i, j in product(range(nrow), range(ncol)):
axs[i, j] = plt.subplot(gs[i, j*3], projection=prj)
caxs[i, j] = plt.subplot(gs[i, j*3+1])
levels = dict(
photoC_TOT_zint_100m=np.arange(-10, 11, 1),
POC_FLUX_100m=np.arange(-4, 4.2, 0.2),
ep_ratio_100m=np.arange(-10, 11, 1)/100.,
NPP_diat_frac_100m=np.arange(-0.75, 0.76, 0.1)
)
cmap_field = cmocean.cm.balance
for n, field in enumerate(['photoC_TOT_zint_100m', 'POC_FLUX_100m', 'ep_ratio_100m', 'NPP_diat_frac_100m']):
i, j = np.unravel_index(n, axs.shape)
ax = axs[i, j]
cf = ax.contourf(
dsp.TLONG, dsp.TLAT, dsp[field],
levels=levels[field],
extend='both',
cmap=cmap_field,
norm=colors.BoundaryNorm(levels[field], ncolors=cmap_field.N),
transform=ccrs.PlateCarree(),
)
land = ax.add_feature(
cartopy.feature.NaturalEarthFeature(
'physical','land','110m',
edgecolor='face',
facecolor='gray'
)
)
ax.set_title(titles[field]) #dsp[field].attrs['title_str'])
cb = plt.colorbar(cf, cax=caxs[i, j])
if 'units' in dsp[field].attrs:
cb.ax.set_title(dsp[field].attrs['units'])
utils.label_plots(fig, [ax for ax in axs.ravel()], xoff=0.02, yoff=0)
utils.savefig('bio-pump-change-2100-SSP5-8.5.pdf')
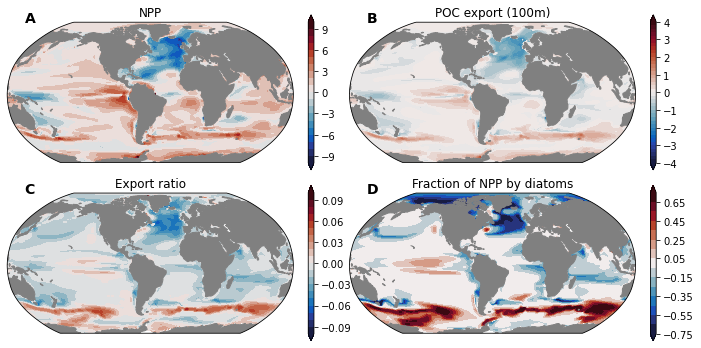
del client
del cluster